The best way to learn about coding is to write your own programs. Let’s start by making the shortest possible program in Processing. It will demonstrate how coding works by drawing a line on the screen.
Open Processing’s IDE and click this icon to create a new program and write the following code:
line(0, 0, 100, 100);
Run the program by pressing the run icon: What you should see on the screen now is this:
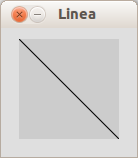
We only use one single command here:
line(x1, y1, x2, y2)
: Draws a line from the coordinate (x1, y1) to (x2, y2). In this example from the top left corner (0,0) to the bottom right corner (100,100). The window it is drawn in is 100 × 100 pixels big.
What you need to know:
- You need to end every statement with a semicolon, ‘;’. If you forget to place it, the code will neither compile nor run.
- By default, windows for Processing programs are only 100×100 pixels with a gray background. The default drawing color is black. Of course, each of these settings can be changed.
Now let’s write a program that draws two differently colored lines on a white background:
background(255); line(0, 0, 100, 100); stroke(0, 0, 255); line(0, 100, 100, 0);
New commands used here:
background(gray)
: Sets the background color from 0 – black, to 255 – white. You can also usebackround(red, green, blue)
to set the background color to any color you want.stroke(red, green, blue)
: Sets the stroke color. Each color value can be from 0 to 255. In this case the stroke color is blue since red=0, green=0 and blue=255.
When you run the program, what you should see on the screen is this:
Your programs will always execute from top-down, statement-by-statement. The first command of the code sets the background color to white. The next command draws a line using the default color (black) from coordinate (0,0) to (100,100). Then the stroke color is changed to blue, after which it draws the second line from the bottom left corner (0,100) to the top right corner (100,0).

Experiment further
- Add more lines in different colors and change the background color.
- Change the order of the program. For example, what happens if you change the background color in the last command instead?