The types of program we’ve done so far are called static programs. This means that it never changes. This is because it runs only once and when it reaches the last line of code, it stops. If we want the program to be interactive we need to allow input while the program runs continuously in a loop.
With Processing, there are two functions you need in order to create a dynamic program. Those functions are setup()
and draw()
.
setup()
runs only once and is where we initialize or set values only once the program. draw()
runs in a loop over and over again until the program is stopped.
Lets write a program that draws a line again but this time using the new functions:
void setup() { size(300, 300); } void draw() { line(0 ,0, width, height); }
setup()
: the code within its curly brackets runs only once when the program starts. Since we need to set the size of the window only once, this is where we do so.draw()
: the code within its curly brackets runs in a loop. It is executed line-by-line, top-down until it reaches the last line. It then repeats by going back to the top of the function and running again.
This programs first sets the window size to 300 x 300 pixel and then repeatedly draws a line. Because it’s drawing the same line, you actually won’t see any changes.
Let’s write another program to make this visible.
void setup() { size(300,300); } void draw() { line(0, 0, mouseX, mouseY); }
mouseX
: The X coordinate of the mouse pointer.mouseY
: The Y coordinate of the mouse pointer.
This program allows you to interact with the screen by moving the mouse as shown in the following image. Inside draw(),
the command line()
draws a line from (0,0) to your mouse pointer. It repeats over and over, so as you move your mouse, a new line is draw from the origin to your mouse pointer.
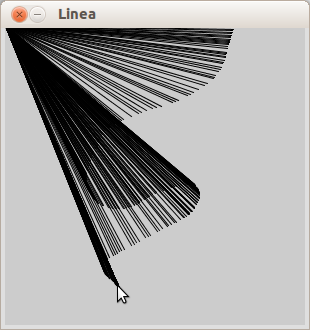
As you can see, you’re leaving a trace of lines when you move the mouse. This is because each line that is drawn is never removed or is drawn over by something else. What do you think will happen when you run the following program?
void setup() { size(300,300); } void draw() { background(255); line(0, 0, mouseX, mouseY); }
We’ve added a line of code in the beginning of draw()
; the command background()
sets the background to white. When you run the program, you’ll notice that there is no longer a trail – that there is only one line drawn from the origin to your mouse pointer.
As explained earlier, each time draw()
reaches the last line, it runs again from the top. Thus, it will redraw the entire background to white, and the line that was drawn previously is effectively erased. A new line is then drawn. Over and over again, the line is drawn and then erased.