As you have learned previously, digital signals only have two opposite states: 1 or 0. For example, if you push a button, its state changes from one to the other; a LED is either on or off.
The real world is not digital. Water for example is not just hot or cold, it can be lukewarm. To be able to measure that, and other things in the real world, we can’t use digital signals. Instead we use Analog signals.
Instead of 2 opposite states, Analog signals has continuous levels. So if you use a light sensor, you can get many different values telling you how much light there is in the room, instead of a simple dark/bright. Or if it’s a thermometer, it’ll tell you the temperature in number, instead of cold/hot.
With Arduino, you can get analog values from the analog pins. You can see a group of pins on your board marked as ANALOG IN, which are named from A0 to A5. Instead of reading just 0V or 5V these pins let you read 1024 values in between. 0V has a reading of 0; 2.5V has a reading of 512; and 5V has a reading of 1023. To read values from an analog pin you need to use the function analogRead()
, instead of digitalRead()
.
To further explain analog signals, we need to introduce the potentiometer. A potentiometer is a knob that you can turn to control something, e.g., the volume controller on your stereo is a potentiometer. When the two outer pins of potentiometer are connected to GND and 5V respectively, you can use the knob to control how much voltage is applied to the middle pin – the range being 0V to 5V.
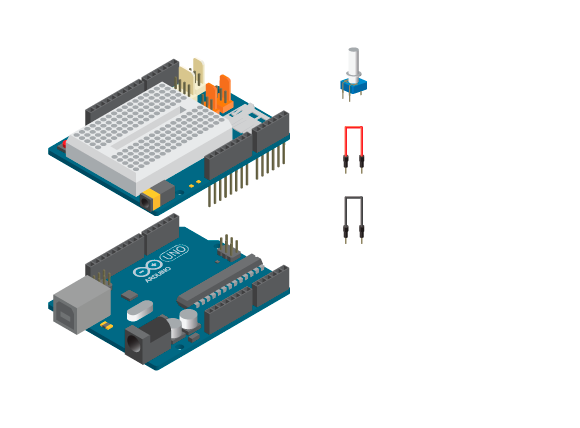
Here we’re going to experiment a bit and use a potentiometer to control the blinking of a LED. Connect the middle pint to analog pin A5 and then connect the other outer pins to 5V and GND. We will use the on-board LED as output.
int ledPin = 13; void setup() { pinMode(ledPin, OUTPUT); } void loop() { int val = analogRead(A5); digitalWrite(ledPin, HIGH); delay(val); digitalWrite(ledPin, LOW); delay(val); }
You can find a new command:
analogRead(pinNumber)
: This command takes the reading from an analog pin specified by thepinNumber
, which can be A0 to A5. Its value ranges from 0 (0V) to 1023 (5V).- The analog pins can only be used as inputs so there is no need to declair the pin mode in
setup()
.
The reading from potentiometer connected to analog pin A5 will change the delay time and therefore the blinking speed of on-board LED on pin 13. When you turn the potentiometer knob, you can see a change in the blinking frequency.
Experiment Further
- The
analogRead()
value has 1024 levels – between 0 and 1023. Can you find a way to process the value, so there’re fewer levels? Say we need only ten different levels. We want to keep 0V as 0 and 5V as 10, rather than 1023. (HINT: Look up a function calledmap()
.) - What happens if you reduce the number of levels of
analogRead()
to only two – effectively 0 and 1? Try applying it to the digital button example. Do you feel you’ve fully grasped the connection between analog and digital signals?