When using two servos at the same time, your Arduino board will have a problem distributing enough current. There are different techniques to overcome this. Here you will explore how to write signals to the servos separately through the program.
Attach two servos to the shield, one to D9 and the other one to D10.
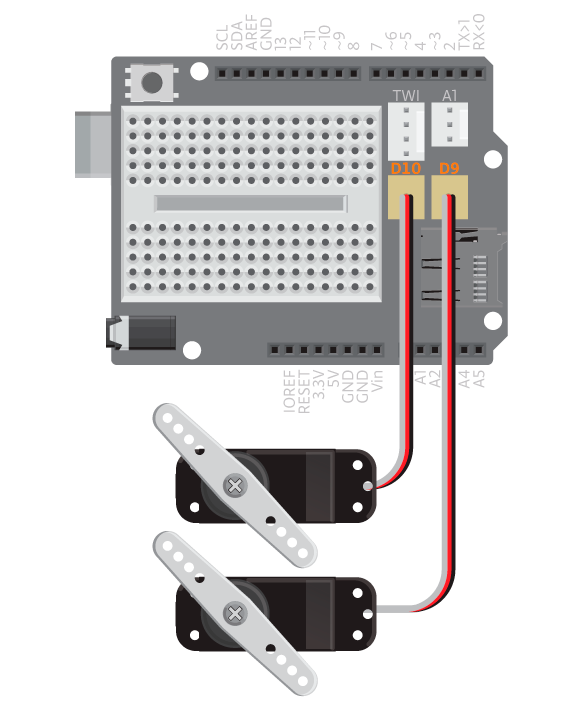
#include <Servo.h> Servo myservo1, myservo2; void setup() { myservo1.attach(9); myservo2.attach(10); } void loop() { myservo2.detach(); myservo1.attach(9); myservo1.write(70); delay(1000); myservo1.write(120); delay(1000); myservo1.detach(); myservo2.attach(10); myservo2.write(70); delay(1000); myservo2.write(120); delay(1000); }
The program connects myservo2
and spints it to an angle of 70 degrees, waits 1 second, and then turns the same engine to 120 degrees.
Then it disconnects myservo2
and connects myservo1
and repeats the process.
It will alternate thereafter.
Experiment further
- Create a robot that uses a continuous rotation servo and a standard rotation servo to move forward. How would you do this?